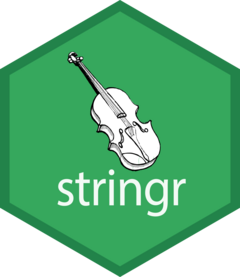
Function reference
-
str_count()
- Count number of matches
-
str_detect()
- Detect the presence/absence of a match
-
str_escape()
- Escape regular expression metacharacters
-
str_extract()
str_extract_all()
- Extract the complete match
-
str_locate()
str_locate_all()
- Find location of match
-
str_match()
str_match_all()
- Extract components (capturing groups) from a match
-
str_replace()
str_replace_all()
- Replace matches with new text
-
str_remove()
str_remove_all()
- Remove matched patterns
-
str_split()
str_split_1()
str_split_fixed()
str_split_i()
- Split up a string into pieces
-
str_starts()
str_ends()
- Detect the presence/absence of a match at the start/end
-
fixed()
coll()
regex()
boundary()
- Control matching behaviour with modifier functions
Vector
Unlike other pattern matching functions, these functions operate on the original character vector, not the individual matches.
-
str_subset()
- Find matching elements
-
str_which()
- Find matching indices
-
str_c()
- Join multiple strings into one string
-
str_flatten()
str_flatten_comma()
- Flatten a string
-
str_glue()
str_glue_data()
- Interpolation with glue
-
str_dup()
- Duplicate a string
-
str_length()
str_width()
- Compute the length/width
-
str_pad()
- Pad a string to minimum width
-
str_sub()
`str_sub<-`()
str_sub_all()
- Get and set substrings using their positions
-
str_trim()
str_squish()
- Remove whitespace
-
str_trunc()
- Truncate a string to maximum width
-
str_wrap()
- Wrap words into nicely formatted paragraphs
-
str_order()
str_rank()
str_sort()
- Order, rank, or sort a character vector
-
str_equal()
- Determine if two strings are equivalent
-
str_to_upper()
str_to_lower()
str_to_title()
str_to_sentence()
- Convert string to upper case, lower case, title case, or sentence case
-
str_unique()
- Remove duplicated strings
-
invert_match()
- Switch location of matches to location of non-matches
-
str_conv()
- Specify the encoding of a string
-
str_like()
- Detect a pattern in the same way as
SQL
'sLIKE
operator
-
str_replace_na()
- Turn NA into "NA"
-
str_view()
- View strings and matches
-
word()
- Extract words from a sentence